Hello all!
I've been working on a Fallout 1/2 map viewer, but I've run into a bit of an issue rendering objects.
Here's a screenshot of my viewer (with a hex grid overlay on):
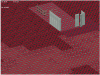
and here's the same map in Mapper2:
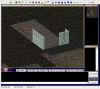
As you can see, some of the objects are rendered at the wrong position. What I do is take the screen position from the hex position of the object, and then subtract width/2 and height from it, and then add the FRM offsets. For the most part I've been working off of ancient FIFE engine code, because it's the only readable source I could find.
Does anyone have any source code for positioning/drawing Fallout maps I could use? I would greatly appreciate it.
Thanks
I've been working on a Fallout 1/2 map viewer, but I've run into a bit of an issue rendering objects.
Here's a screenshot of my viewer (with a hex grid overlay on):
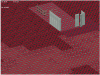
and here's the same map in Mapper2:
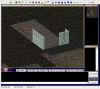
As you can see, some of the objects are rendered at the wrong position. What I do is take the screen position from the hex position of the object, and then subtract width/2 and height from it, and then add the FRM offsets. For the most part I've been working off of ancient FIFE engine code, because it's the only readable source I could find.
Does anyone have any source code for positioning/drawing Fallout maps I could use? I would greatly appreciate it.
Thanks