I thought I'd stop dumping everything in my ill-named define.h thread, and make a dedicated thread.
I came to the conclusion a short while ago that much of my megalomania is outside of the scope of the regular game, and would probably result in breaking it completely. So, I want to try and introduce a lot of that megalomania into the virgin soil that is random encounters.
But enough about me, what can this do for YOU, the humble, non-megalomanic modder? A lot! I hope! Eventually! Introduce new types of critters, weapons and scenery without having to finnick around with worldmap.txt, that horrible thing. You could also make everything a lot more varied by shuffling up the maps, critters, everything considerably (more on that in a bit).
Now, what would we lose in the process?
- as far as I know, there's no way to implement the outdoorsman check. I never was terribly fond of the mechanic (I don't have to run away from rats manually, but just need to click a button), but you might disagree.
- map type (desert, mountain, etc.) becomes harder to pin down based on the world map, unless you were to go through the effort of micro-allocating everything like in the worldmap.txt.
Now for my latest trick: constructing map templates with an array constructed from the debug text!
What this does is allow you to denote a rectangle on the map you're at (I prefer to use two keystrokes, one for upper left corner, one for size based on distance from upper left corner), within which every piece of scenery and every wall is saved in an array (you can also copy critters of course if you want). When I say saved, I mean it's printed in the debug log through debug_list_comprehensive:
Suppose I want to copy this part of a building:
all I do is make the rectangle around it, then check my debug log where I find it all stored as a lovely array, which I just need to assign to a variable:
Now I can place it wherever I want:
Sadly you can't do this copying with roofs and tiles (and probably never will, right @phobos2077?) because there's no way to place them through scripts, so any maps you piece together this way won't ever look very good (especially where cities are concerned: no curbs and stuff), but it should allow you to do some interesting stuff.
Next up I'll show something I've been doing with randomized containers along walls that might be interesting as well.
BTW, I just put up the example script segments to show a bit of where I'm coming from, I'll release proper sources once I've got something finalized.
edit: hmm, seems I cheered too soon, it appears to work properly only about half the time, with the rest of the time the north facing wall getting garbled up... I need to look into fallout's tile numbering a bit more again.
edit2: oh right, forgot to account for uneven sizes
I came to the conclusion a short while ago that much of my megalomania is outside of the scope of the regular game, and would probably result in breaking it completely. So, I want to try and introduce a lot of that megalomania into the virgin soil that is random encounters.
But enough about me, what can this do for YOU, the humble, non-megalomanic modder? A lot! I hope! Eventually! Introduce new types of critters, weapons and scenery without having to finnick around with worldmap.txt, that horrible thing. You could also make everything a lot more varied by shuffling up the maps, critters, everything considerably (more on that in a bit).
Now, what would we lose in the process?
- as far as I know, there's no way to implement the outdoorsman check. I never was terribly fond of the mechanic (I don't have to run away from rats manually, but just need to click a button), but you might disagree.
- map type (desert, mountain, etc.) becomes harder to pin down based on the world map, unless you were to go through the effort of micro-allocating everything like in the worldmap.txt.
Now for my latest trick: constructing map templates with an array constructed from the debug text!
Code:
procedure build_rectangle_from_text(variable upper_left, variable array) begin
variable min,max,tile,north_corner,border_corners,east_corner,west_corner,upper_right,lower_left,lower_right,corners,temp_upper_right;
variable obj,tile_contents,tile_counter,scenery_array,objs,size;
size:=get_array(array, len_array(array) - 1);
temp_upper_right:=tile_num_in_direction(upper_left, 1, size);
upper_right:=tile_num_in_direction(temp_upper_right, 5, size/2);
lower_left:=tile_num_in_direction(upper_left, 2, size);
lower_right:=tile_num_in_direction(upper_right, 2, size);
corners:=[upper_left, upper_right, lower_left, lower_right];
min:=array_min(corners)-50;
max:=array_max(corners)+50;
//tile:=min;
tile_counter:=0;
while tile <= max and tile_counter < len_array(array) - 1 do begin
//display_msg("f " + len_array(tile_get_objs(tile, dude_elevation)));
if tile_in_tile_rect(upper_left, upper_right, lower_left, lower_right, tile) then begin //upper left, upper right, lower left, lower right, tile
if array[tile_counter] > 0 then
create_object(array[tile_counter], tile, dude_elevation);
tile_counter+=1;
end
tile+=1;
end
end
procedure get_scenery_in_rectangle(variable upper_left, variable size) begin
variable min,max,tile,north_corner,border_corners,east_corner,west_corner,upper_right,lower_left,lower_right,corners,temp_upper_right;
variable obj,tile_contents,tile_counter,scenery_array,objs;
temp_upper_right:=tile_num_in_direction(upper_left, 1, size);
upper_right:=tile_num_in_direction(temp_upper_right, 5, size/2);
lower_left:=tile_num_in_direction(upper_left, 2, size);
lower_right:=tile_num_in_direction(upper_right, 2, size);
//create_object(PID_BOOKCASE_2HEX_LEFT_LIGHT, upper_left, dude_elevation);
//create_object(PID_BOOKCASE_2HEX_LEFT_LIGHT, upper_right, dude_elevation);
//create_object(PID_BOOKCASE_2HEX_LEFT_LIGHT, lower_left, dude_elevation);
//create_object(PID_BOOKCASE_2HEX_LEFT_LIGHT, lower_right, dude_elevation);
corners:=[upper_left, upper_right, lower_left, lower_right];
min:=array_min(corners)-50;
max:=array_max(corners)+50;
tile:=min;
tile_counter:=0;
scenery_array:=create_array(0,0);
while tile <= max do begin
//display_msg("f " + len_array(tile_get_objs(tile, dude_elevation)));
if tile_in_tile_rect(upper_left, upper_right, lower_left, lower_right, tile) then begin //upper left, upper right, lower left, lower right, tile
objs:=tile_get_objs(tile, dude_elevation);
tile_contents:=0;
foreach obj in objs begin
if (obj_type(obj) == OBJ_TYPE_WALL) or (obj_type(obj) == OBJ_TYPE_SCENERY) or (obj_type(obj) == OBJ_TYPE_TILE) or (obj_type(obj) == OBJ_TYPE_ITEM) then begin
display_msg(obj_name(obj) + obj_type(obj));
tile_contents:=obj_pid(obj);
end
end
call array_push_no_return(scenery_array, tile_contents);
//create_object(PID_10MM_PISTOL, tile, dude_elevation);
end
tile+=1;
tile_counter+=1;
end
call array_push_no_return(scenery_array, size); //last value in array denotes size
call debug_list_comprehensive(scenery_array);
//debug_msg("" + len_array(scenery_array) + debug_array_str(scenery_array));
end
What this does is allow you to denote a rectangle on the map you're at (I prefer to use two keystrokes, one for upper left corner, one for size based on distance from upper left corner), within which every piece of scenery and every wall is saved in an array (you can also copy critters of course if you want). When I say saved, I mean it's printed in the debug log through debug_list_comprehensive:
Code:
procedure debug_list_comprehensive(variable arr) begin
variable i := 0, x:=0, k, s, len;
len := len_array(arr);
while x < len do begin
x+=10;
s := "";
while i < x and i < len do begin
s += arr[i];
if i < len - 1 then
s += ", ";
i++;
end
if x == 10 then
debug_msg("[" + s);
else if x < len then
debug_msg(s);
else
debug_msg(s + "]");
end
end
Suppose I want to copy this part of a building:
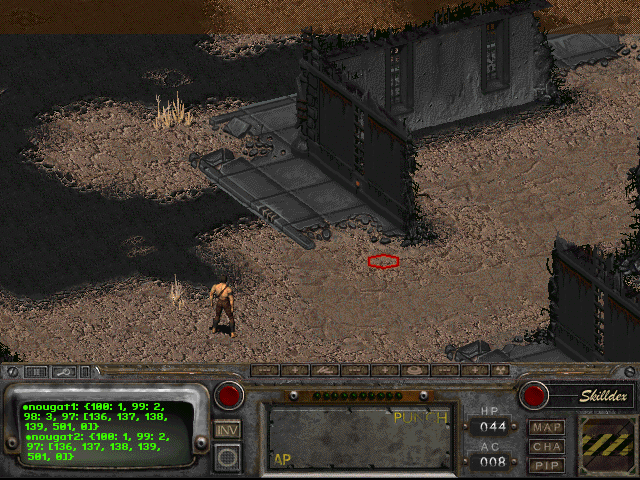
all I do is make the rectangle around it, then check my debug log where I find it all stored as a lovely array, which I just need to assign to a variable:
Code:
test_array:=[0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 50332280, 50332238, 50332293, 50332236, 50332237, 50332239, 50332292,
50332238, 50332234, 50332236, 50332269, 0, 0, 0, 0, 50332270, 0,
50332270, 0, 50332270, 0, 50332270, 0, 50332270, 50332162, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 50332177, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 50332178, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 50332288,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 50332177, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 50332178, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 50332273, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
13];
Now I can place it wherever I want:
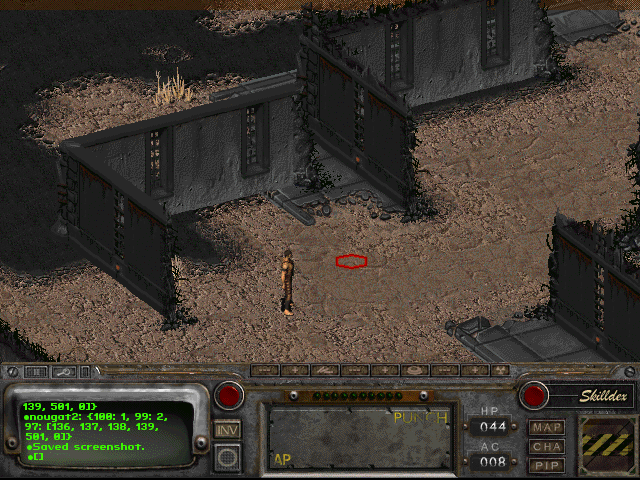
Sadly you can't do this copying with roofs and tiles (and probably never will, right @phobos2077?) because there's no way to place them through scripts, so any maps you piece together this way won't ever look very good (especially where cities are concerned: no curbs and stuff), but it should allow you to do some interesting stuff.
Next up I'll show something I've been doing with randomized containers along walls that might be interesting as well.
BTW, I just put up the example script segments to show a bit of where I'm coming from, I'll release proper sources once I've got something finalized.
edit: hmm, seems I cheered too soon, it appears to work properly only about half the time, with the rest of the time the north facing wall getting garbled up... I need to look into fallout's tile numbering a bit more again.
edit2: oh right, forgot to account for uneven sizes
Last edited: