
The standard/old school way is to modify their proto files (00000470.pro and 00000480.pro) and related scripts (ccturret, qcturret and wcturret). Using sfall global/hook scripts makes things simpler and is also more expandable, plus it's fun to make my own personal mini mod.

1. Changing action flags in proto files with a global script:
Code:
// gl_loot_bots.ssl
procedure start;
#include ".\HEADERS\DEFINE.H"
#include ".\HEADERS\define_extra.h"
procedure start begin
if(game_loaded) then begin
set_global_script_repeat(60);
end else begin
// Auto-Cannon (for some reason its PID is not defined in CRITRPID.H)
set_proto_data(16777696, PROTO_CR_ACTION_FLAGS, (CFLG_NODROP + CFLG_NOLIMBS + CFLG_NOAGES + CFLG_NOHEAL + CFLG_FLATTN + CFLG_NOKNOCKDOWN));
// Gun Turret
set_proto_data(PID_AUTO_GAT_GUN, PROTO_CR_ACTION_FLAGS, (CFLG_NODROP + CFLG_NOLIMBS + CFLG_NOAGES + CFLG_NOHEAL + CFLG_FLATTN + CFLG_NOKNOCKDOWN));
end
end
Here's my half-a**ed explanation of those CFLG_* in set_proto_data. If you open their proto files in hex editor, it looks like this:
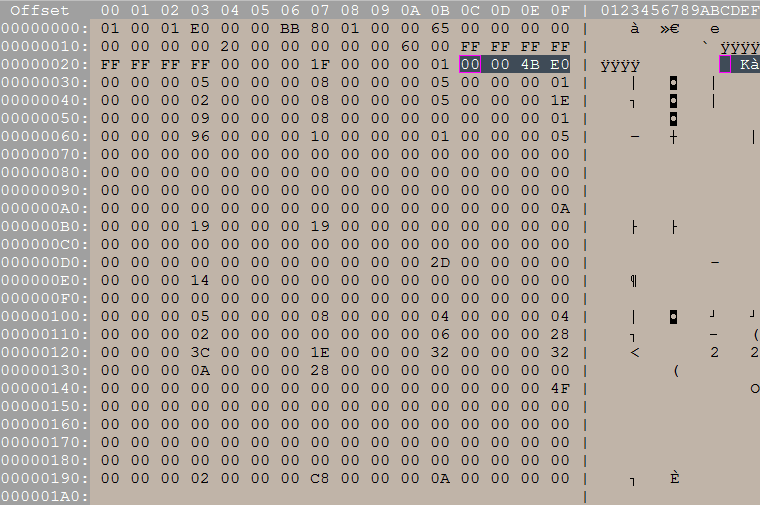
The selected part contains the action flags for critters. From define_extra.h or the article on Vault-Tec Labs, the description of action flags is:
Code:
#define CFLG_BARTER 2 //0x00000002 - Barter (can trade with)
#define CFLG_NOSTEAL 32 //0x00000020 - Steal (cannot steal from)
#define CFLG_NODROP 64 //0x00000040 - Drop (doesn't drop items)
#define CFLG_NOLIMBS 128 //0x00000080 - Limbs (cannot lose limbs)
#define CFLG_NOAGES 256 //0x00000100 - Ages (dead body does not disappear)
#define CFLG_NOHEAL 512 //0x00000200 - Heal (damage is not cured with time)
#define CFLG_INVULN 1024 //0x00000400 - Invulnerable (cannot be hurt)
#define CFLG_FLATTN 2048 //0x00000800 - Flatten (leaves no dead body)
#define CFLG_SPECIAL 4096 //0x00001000 - Special (there is a special type of death)
#define CFLG_RANGED 8192 //0x00002000 - Range (melee attack is possible at a distance)
#define CFLG_NOKNOCKDOWN 16384 //0x00004000 - Knock (cannot be knocked down)
The value 0x00004BE0 can be expressed as the sum of 0x00004000, 0x00000800, 0x00000200, 0x00000100, 0x00000080, 0x00000040 and 0x00000020. Which means Knock, Flatten, Heal, Ages, Limbs, Drop and Steal flags being set. To make turrets lootable, only Steal flag needs to be removed.
The new value should be (0x00004000 + 0x00000800 + 0x00000200 + 0x00000100 + 0x00000080 + 0x00000040) = 0x00004BC0. But that's only necessary for hex-editing, with sfall scripting we only need to add up the flag variables and leave the rest to the compiler.
(BTW, I hope Cubik2k's FO1/2 Critters Editor can be updated to let us edit those action flags and some other attributes.)
2. Preventing Player from stealing turret weapons:
Now turrets are stealable and lootable, but if you manage to steal from the turrets outside of Sierra Amy Depot or Navarro, you'll find that you can steal their Dual Minigun/Plasma Cannon. Because devs only put the weapon in their inventory not the left hand slot (you can't steal "equipped" items). There are several ways to prevent/fix this. One is editing the maps to put weapons in their left hand slots. Another is editing their scripts to make them automatically equip/wield weapons when you enter the maps, like the turrets on the Enclave Oil Rig. But I'm gonna take the other approach: making them unstealable while they're alive.
sfall 3.5 added hs_useskill hook script, which runs when using any skill on any object. The logic is pretty simple here:
Code:
// hs_useskill.ssl
procedure start;
#include ".\HEADERS\DEFINE.H"
#include ".\HEADERS\define_extra.h"
procedure start begin
variable user, target, skill;
if not init_hook then begin
user := get_sfall_arg;
target := get_sfall_arg;
skill := get_sfall_arg;
if ((user == dude_obj) and (skill == SKILL_STEAL)) then begin
if ((obj_pid(target) == 16777696) or (obj_pid(target) == PID_AUTO_GAT_GUN)) then begin
if not (is_critter_dead(target)) then begin
set_sfall_return(0);
end
end
end
end
end
3. Adding some reasonable loots on dead turrets and cleaning up their NPC-only items:
Again, the standard/old school way is editing their scripts to add destroy_p_proc procedure, which will run when they get killed. Here we will do the same thing with only one script.
sfall 1.46 added hs_ondeath hook script, which runs immediately after a critter dies for any reason. This one is a bit more complex than previous ones:
Code:
//hs_ondeath.ssl
procedure start;
#include ".\HEADERS\DEFINE.H"
#include ".\HEADERS\define_extra.h"
procedure start begin
variable critter, removed_item, ammo, ammo_count;
if not init_hook then begin
critter := get_sfall_arg;
// Auto-Cannon
if (obj_pid(critter) == 16777696) then begin
if (obj_is_carrying_obj_pid(critter, PID_DUAL_MINIGUN)) then begin
if (random(0, 1) == 1) then begin
ammo := create_object(PID_5MM_AP, 0, 0);
ammo_count := random(1, 2);
add_mult_objs_to_inven(critter, ammo, ammo_count);
end else begin
ammo := create_object(PID_5MM_JHP, 0, 0);
ammo_count := random(1, 2);
add_mult_objs_to_inven(critter, ammo, ammo_count);
end
end
if ((obj_is_carrying_obj_pid(critter, PID_HEAVY_DUAL_MINIGUN)) and (random(0, 1) == 1)) then begin
ammo := create_object(PID_223_FMJ, 0, 0);
ammo_count := random(1, 2);
add_mult_objs_to_inven(critter, ammo, ammo_count);
end
while ((obj_is_carrying_obj_pid(critter, PID_DUAL_MINIGUN)) or (obj_is_carrying_obj_pid(critter, PID_HEAVY_DUAL_MINIGUN))) do begin
removed_item := obj_carrying_pid_obj(critter, PID_DUAL_MINIGUN);
rm_obj_from_inven(critter, removed_item);
destroy_object(removed_item);
removed_item := obj_carrying_pid_obj(critter, PID_HEAVY_DUAL_MINIGUN);
rm_obj_from_inven(critter, removed_item);
destroy_object(removed_item);
end
end
// Gun Turret
if (obj_pid(critter) == PID_AUTO_GAT_GUN) then begin
if (obj_is_carrying_obj_pid(critter, PID_GUN_TURRET_WEAPON) and (random(0 ,1) == 1)) then begin
add_obj_to_inven(critter, create_object(PID_MICRO_FUSION_CELL, 0, 0));
end
while (obj_is_carrying_obj_pid(critter, PID_GUN_TURRET_WEAPON)) do begin
removed_item := obj_carrying_pid_obj(critter, PID_GUN_TURRET_WEAPON);
rm_obj_from_inven(critter, removed_item);
destroy_object(removed_item);
end
end
end
end
The turrets outside of Sierra Amy Depot will have 50% chance of dropping one or two boxes of 5mm JHP and 50% chance of dropping one or two boxes of 5mm AP.
The turrets on the Enclave Oil Rig will have 50% chance of dropping one or two boxes of .223 FMJ.
The turrets in Navarro base will have 50% chance of dropping a pack of Micro Fusion Cell.
And no, you can't have their Daul Minigun, Heavy Dual Minigun or Dual Plasma Cannon.
The three sfall scripts can be easily expanded to include sentry bots or other critters. Or you can add some checks against player's repair/science skill to let you strip usable weapons (actually just dropping some normal weapons on them). But currently I run into an interesting issue with checking their Robo Rocket Launcher.
Last edited: