Femic
First time out of the vault
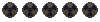
Hi all,
why should I use the keyword export variable and import variable?
Are they any advantages opposite to LVAR MVAR and GVAR?
Let's assume I do this:
Will variable C contain value 99 after importing it?
Thanks and regards.
why should I use the keyword export variable and import variable?
Are they any advantages opposite to LVAR MVAR and GVAR?
Let's assume I do this:
Code:
ScriptA.ssl
export variable C
C := 99;
Code:
ScriptB.ssl
import variable C
Will variable C contain value 99 after importing it?
Thanks and regards.